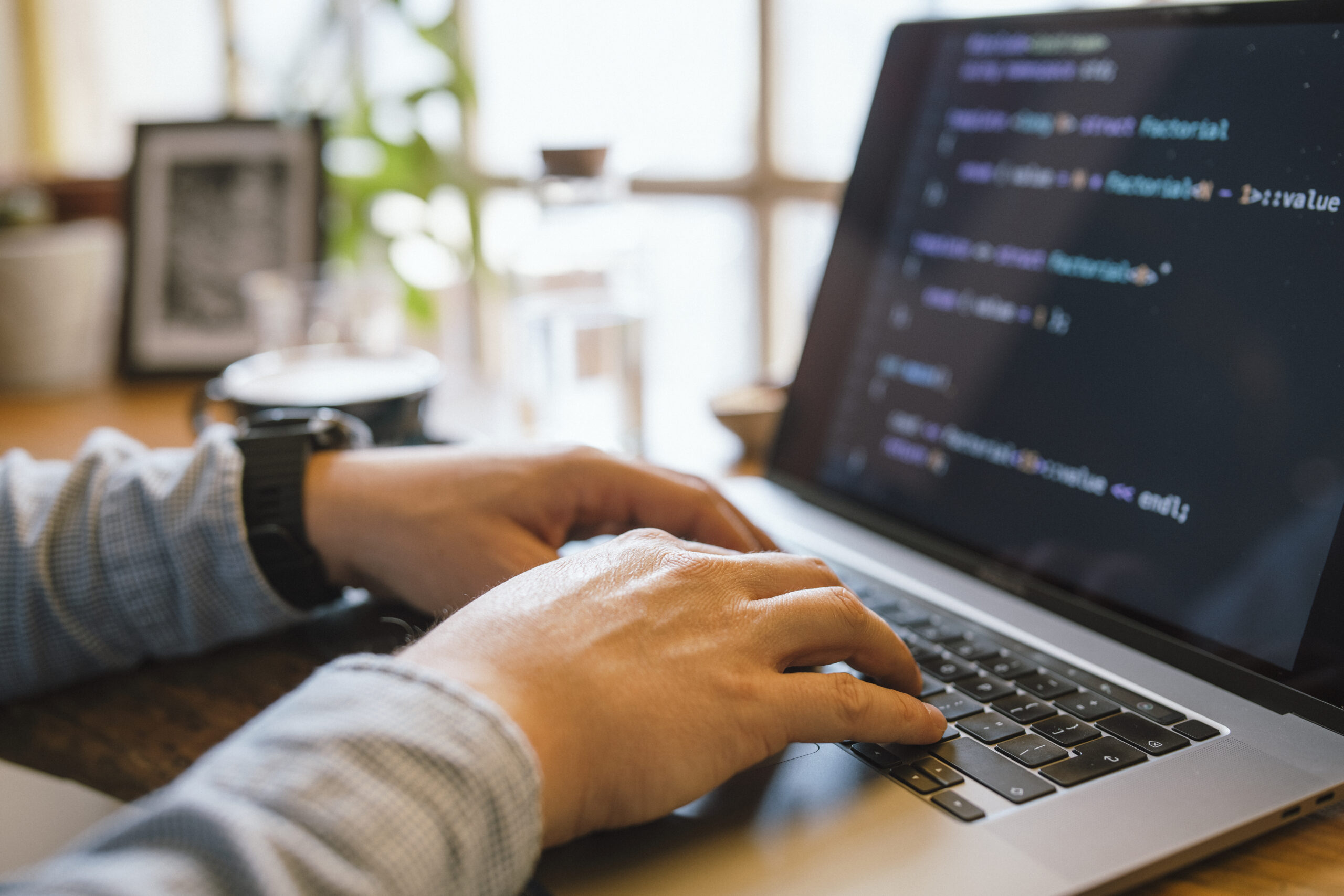
Debugging is Just about the most critical — but typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to solve issues effectively. No matter whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of disappointment and drastically boost your productivity. Listed here are a number of strategies to help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging competencies is by mastering the instruments they use on a daily basis. Even though composing code is a single A part of improvement, knowing how you can connect with it proficiently for the duration of execution is equally important. Modern-day advancement environments come Geared up with strong debugging capabilities — but numerous builders only scratch the floor of what these resources can do.
Choose, by way of example, an Built-in Growth Atmosphere (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments allow you to established breakpoints, inspect the worth of variables at runtime, move as a result of code line by line, and in some cases modify code around the fly. When made use of effectively, they Allow you to notice precisely how your code behaves throughout execution, which happens to be a must have for tracking down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for front-conclude developers. They enable you to inspect the DOM, monitor community requests, perspective actual-time performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can change disheartening UI concerns into workable tasks.
For backend or program-amount builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage in excess of functioning processes and memory management. Discovering these tools could have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with version Manage programs like Git to understand code historical past, obtain the exact second bugs ended up released, and isolate problematic modifications.
Eventually, mastering your instruments means going over and above default options and shortcuts — it’s about acquiring an personal knowledge of your advancement setting so that when concerns occur, you’re not missing at the hours of darkness. The greater you realize your equipment, the more time it is possible to shell out resolving the particular trouble rather than fumbling through the process.
Reproduce the issue
Probably the most crucial — and often overlooked — actions in successful debugging is reproducing the trouble. In advance of leaping to the code or generating guesses, developers need to have to make a regular setting or circumstance the place the bug reliably appears. Without reproducibility, correcting a bug becomes a game of prospect, often leading to wasted time and fragile code changes.
The first step in reproducing a problem is accumulating just as much context as you can. Inquire concerns like: What steps resulted in the issue? Which environment was it in — enhancement, staging, or generation? Are there any logs, screenshots, or mistake messages? The more detail you have, the simpler it becomes to isolate the precise situations under which the bug takes place.
As soon as you’ve collected plenty of details, try to recreate the situation in your local environment. This may suggest inputting the same knowledge, simulating comparable consumer interactions, or mimicking method states. If The difficulty appears intermittently, look at creating automatic tests that replicate the edge conditions or state transitions associated. These tests not simply assist expose the situation but also avert regressions Down the road.
At times, The difficulty might be setting-unique — it might come about only on certain working units, browsers, or below unique configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It necessitates patience, observation, along with a methodical technique. But as you can consistently recreate the bug, you are presently halfway to repairing it. By using a reproducible state of affairs, You should utilize your debugging applications more successfully, check prospective fixes securely, and talk a lot more Obviously using your staff or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Study and Realize the Mistake Messages
Error messages are sometimes the most worthy clues a developer has when a thing goes Erroneous. Rather then observing them as discouraging interruptions, builders need to find out to treat mistake messages as immediate communications through the program. They usually tell you exactly what transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start out by reading through the message carefully As well as in whole. Several developers, particularly when below time pressure, look at the initial line and immediately get started generating assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into engines like google — study and comprehend them initial.
Crack the mistake down into areas. Is it a syntax error, a runtime exception, or a logic mistake? Does it point to a specific file and line range? What module or functionality triggered it? These thoughts can information your investigation and level you toward the liable code.
It’s also handy to comprehend the terminology of the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java frequently abide by predictable designs, and learning to acknowledge these can dramatically increase your debugging method.
Some errors are obscure or generic, and in Those people situations, it’s crucial to look at the context in which the mistake occurred. Test relevant log entries, input values, and recent changes in the codebase.
Don’t ignore compiler or linter warnings both. These typically precede greater concerns and provide hints about probable bugs.
Eventually, error messages usually are not your enemies—they’re your guides. Finding out to interpret them appropriately turns chaos into clarity, assisting you pinpoint challenges speedier, lower debugging time, and become a extra economical and confident developer.
Use Logging Correctly
Logging is One of the more strong applications in the developer’s debugging toolkit. When made use of efficiently, it offers authentic-time insights into how an software behaves, assisting you recognize what’s occurring underneath the hood without needing to pause execution or action throughout the code line by line.
An excellent logging tactic commences with recognizing what to log and check here at what level. Frequent logging stages involve DEBUG, INFO, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic information throughout improvement, INFO for general events (like successful start-ups), WARN for prospective concerns that don’t split the appliance, ERROR for real troubles, and Lethal if the process can’t continue on.
Stay clear of flooding your logs with too much or irrelevant facts. Too much logging can obscure important messages and slow down your procedure. Deal with critical gatherings, point out variations, enter/output values, and critical choice factors inside your code.
Format your log messages Evidently and continuously. Involve context, for instance timestamps, ask for IDs, and performance names, so it’s easier to trace problems in dispersed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
All through debugging, logs Enable you to monitor how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without having halting This system. They’re In particular worthwhile in manufacturing environments where by stepping by way of code isn’t feasible.
Additionally, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
In the long run, smart logging is about balance and clarity. Which has a properly-thought-out logging tactic, it is possible to lessen the time it requires to identify concerns, gain deeper visibility into your applications, and Enhance the Over-all maintainability and reliability of the code.
Think Just like a Detective
Debugging is not just a specialized endeavor—it's a sort of investigation. To effectively recognize and take care of bugs, developers ought to method the method similar to a detective solving a thriller. This way of thinking assists stop working elaborate difficulties into workable components and comply with clues logically to uncover the foundation cause.
Commence by collecting proof. Look at the symptoms of the challenge: mistake messages, incorrect output, or overall performance troubles. Identical to a detective surveys a criminal offense scene, acquire just as much applicable information as you are able to with no jumping to conclusions. Use logs, examination situations, and person experiences to piece alongside one another a clear picture of what’s happening.
Subsequent, sort hypotheses. Check with yourself: What might be creating this conduct? Have any modifications not too long ago been produced towards the codebase? Has this problem occurred before under identical instances? The target will be to slim down opportunities and recognize possible culprits.
Then, test your theories systematically. Try to recreate the problem in a managed natural environment. In case you suspect a certain perform or component, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and let the effects direct you nearer to the truth.
Pay shut consideration to tiny particulars. Bugs normally conceal in the the very least anticipated places—just like a missing semicolon, an off-by-just one error, or a race ailment. Be extensive and affected person, resisting the urge to patch The difficulty devoid of completely understanding it. Momentary fixes could disguise the real trouble, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for upcoming concerns and assistance Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical expertise, tactic problems methodically, and turn into more practical at uncovering hidden concerns in elaborate techniques.
Produce Checks
Creating exams is among the simplest methods to increase your debugging competencies and General growth performance. Checks don't just help catch bugs early but in addition function a security Web that offers you assurance when creating modifications to the codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint precisely in which and when a difficulty happens.
Get started with device checks, which deal with individual capabilities or modules. These compact, isolated checks can immediately expose whether a specific bit of logic is Doing the job as envisioned. Any time a exam fails, you straight away know where to look, significantly reducing some time used debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear right after previously being preset.
Future, combine integration exams and stop-to-stop tests into your workflow. These help ensure that many portions of your application function together effortlessly. They’re notably beneficial for catching bugs that occur in complex units with many elements or services interacting. If a thing breaks, your exams can tell you which Component of the pipeline failed and under what ailments.
Creating checks also forces you to Believe critically regarding your code. To check a function thoroughly, you may need to know its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally sales opportunities to better code structure and less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a powerful initial step. As soon as the examination fails consistently, it is possible to focus on repairing the bug and check out your check move when The difficulty is settled. This tactic ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from a aggravating guessing video game into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a difficult challenge, it’s quick to become immersed in the trouble—observing your monitor for several hours, attempting Remedy immediately after Alternative. But Probably the most underrated debugging resources is actually stepping absent. Getting breaks assists you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
If you're far too near the code for much too extensive, cognitive exhaustion sets in. You might begin overlooking apparent errors or misreading code that you simply wrote just hours earlier. Within this state, your brain becomes less efficient at problem-resolving. A brief wander, a espresso break, or perhaps switching to a special activity for 10–15 minutes can refresh your target. Several developers report locating the basis of a challenge when they've taken time for you to disconnect, allowing their subconscious operate from the qualifications.
Breaks also enable avert burnout, In particular during for a longer period debugging classes. Sitting in front of a screen, mentally trapped, is don't just unproductive and also draining. Stepping away permits you to return with renewed Power along with a clearer state of mind. You might quickly recognize a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you ahead of.
Should you’re trapped, a superb rule of thumb should be to established a timer—debug actively for 45–60 minutes, then have a five–ten minute crack. Use that point to move all over, stretch, or do a little something unrelated to code. It could really feel counterintuitive, Primarily beneath tight deadlines, nonetheless it really causes quicker and more practical debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and aids you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Understand From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It can be a possibility to develop being a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring your self several essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code evaluations, or logging? The answers usually reveal blind spots within your workflow or comprehension and allow you to Create more robust coding practices transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Eventually, you’ll begin to see designs—recurring concerns or frequent errors—that you could proactively avoid.
In team environments, sharing Anything you've figured out from a bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, aiding Other people steer clear of the very same problem boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial parts of your progress journey. In any case, a lot of the ideal developers will not be the ones who publish ideal code, but people that constantly master from their blunders.
Eventually, Each and every bug you deal with adds a different layer to your ability established. So subsequent time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — though the payoff is huge. It can make you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become much better at That which you do.